I was searching a Facebook application example with CodeIgniter
today. There weren’t many tutorials on it so decided to give a try for
FB application with CI. So let’s see what and how I did it.
I’m assuming you know how to setup a FB application. If you don’t then please visit here, this is a great step-by-step process to start a FB application.
Well, if you are done setting up the process according to the instruction, you supposed to have few things in your hand: an API key, Secret key, Callback URL and base URL.
Now you need to Download the latest version of CodeIgniter and unzip the folder in your Base URL location. Set base URL from application/config/config.php
Example : $config['base_url'] = “http://apps.facebook.com/your-app-name/”;
Ok, now set your default controller from here: application/config/routes.php
Default controller is “welcome” – for sample application I didn’t change it. I just worked on default controller. You can change it according to your needs. If you want to use different controller keeping default controller as it is then add that controller name to your Callback URL.
Now let’s download the Facebook client library from here:
Once you have this package in your hand place them in your application/plugins (if there is not folder named plugins then create it) folder. Note that, you can also add the library in the library folder.There are only three files of FB client library- place them in your plugins folder. But don’t forget to rename your facebook.php to facebook_pi.php
Yay ! We are done all initial setting stuffs. Now need to see some actions!
Open you default controller (in my case “Welcome” controller).
First of all we need to create a __Contrust class so that it gets initiated for the first time application user.
So our construct class will be look like this:
So we have called Facebook API key and secret key and then we loaded
the client library that we added as plugin in plugins folder. At the end
we checked if the user is logged in or not. Well, you can avoid it by
defining a Session key. But I found it was easier to avoid Session key
problem with the application by allowing users logged in first.
We supposed to get connected with FB API by this time. So let’s play with FB API now. Create a function called index () and lets retrieve logged in user’s all friends from it.
So it should look like this
So we got our friends list in “friends” array. Let’s go to
“welcome_message” view in view folder to retrieve and show all friends
that the array is containing. My view looks like this bellow.
Two facebook tags are added here. One is for Profile picture of my
friends and second tag is to show my friends name. I have also added
three more CSS class to show it a little bit better.
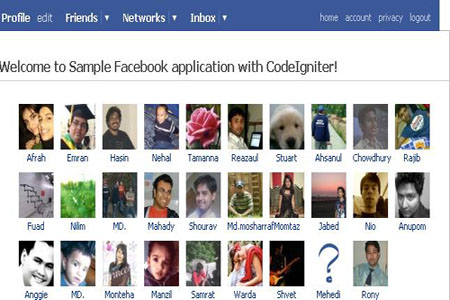
They are:
You can add this at the top of your view page in the <style> tag.
Now let’s go to the FB canvas and see what is my page is showing. From the above example my canvas page shows the output like this:
Okay, if you want to add database, you just need to configure your application/config/databse.php file. Rest of the things are same.
Ahh ! It’s cool. I guess if I use any FW for FB application first I will check the volume of the application. I think there is no meaning to use FW for a small FB application.
I’m assuming you know how to setup a FB application. If you don’t then please visit here, this is a great step-by-step process to start a FB application.
Well, if you are done setting up the process according to the instruction, you supposed to have few things in your hand: an API key, Secret key, Callback URL and base URL.
Now you need to Download the latest version of CodeIgniter and unzip the folder in your Base URL location. Set base URL from application/config/config.php
Example : $config['base_url'] = “http://apps.facebook.com/your-app-name/”;
Ok, now set your default controller from here: application/config/routes.php
Default controller is “welcome” – for sample application I didn’t change it. I just worked on default controller. You can change it according to your needs. If you want to use different controller keeping default controller as it is then add that controller name to your Callback URL.
Now let’s download the Facebook client library from here:
Once you have this package in your hand place them in your application/plugins (if there is not folder named plugins then create it) folder. Note that, you can also add the library in the library folder.There are only three files of FB client library- place them in your plugins folder. But don’t forget to rename your facebook.php to facebook_pi.php
Yay ! We are done all initial setting stuffs. Now need to see some actions!
Open you default controller (in my case “Welcome” controller).
First of all we need to create a __Contrust class so that it gets initiated for the first time application user.
So our construct class will be look like this:
01 | class Welcome extends Controller { |
02 | var $facebook ; |
03 | //API and secret key that you got from your application setup. |
04 | var $__fbApiKey = 'YourKey' ; |
05 | var $__fbSecret = 'YourSecretKey' ; |
06 |
07 | function __construct() |
08 | { |
09 | parent::__construct(); |
10 | //load the client library that we added as plugin in plugins folder. |
11 | $this ->load->plugin( 'facebook' ); |
12 | // Prevent the 'Undefined index: facebook_config' notice from being thrown. |
13 | $GLOBALS [ 'facebook_config' ][ 'debug' ] = NULL; |
14 | // Create a Facebook client API object. |
15 | $this ->facebook = new Facebook( $this ->__fbApiKey, $this ->__fbSecret); |
16 | $user = $this ->facebook->require_login(); |
17 | } |
18 | function Welcome() { |
19 | parent::Controller(); |
20 | } |
21 | // You should place your Controller's methods below. |
22 | function index() |
23 | { |
24 | // Retrieve the user's friends and pass them to the view. |
25 | $friends = $this ->facebook->api_client->friends_get(); |
26 | $this ->load->view( 'welcome_message' , array ( "friends" => $friends )); |
27 | } |
We supposed to get connected with FB API by this time. So let’s play with FB API now. Create a function called index () and lets retrieve logged in user’s all friends from it.
So it should look like this
1 | function index() |
2 | { |
3 | // Retrieve the user's friends and pass them to the view. |
4 | $friends = $this ->facebook->api_client->friends_get(); |
5 | $this ->load->view( 'welcome_message' , array ( "friends" , $friends )); |
6 | } |
01 | < h3 >Welcome to Sample Facebook application with CodeIgniter!</ h3 > |
02 | < div class = "container" > |
03 | < ? php |
04 | foreach ($friends as $id) |
05 | { |
06 | ?> |
07 | < div class = 'img' > |
08 | < fb :profile-pic uid='<?=$id?>' firstnameonly = 'true' linked='true' size='square'/> |
09 | < div class = 'name' > |
10 | < fb :name uid='<?=$id?>' firstnameonly = 'true' capitalize='true' useyou = 'false'/> |
11 | </ fb ></ div > |
12 | </ fb ></ div > |
13 | < ? php |
14 | } |
15 | ?></ div > |
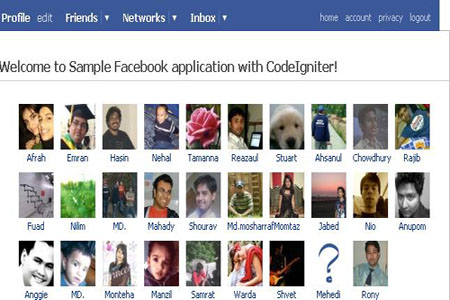
They are:
01 | .img { |
02 | float : left ; |
03 | padding : 10px 0px 0px 10px ; |
04 | width : 50px ; |
05 | overflow : visible ; |
06 | } |
07 |
08 | .name { |
09 | padding-top : 0px ; |
10 | text-align : center ; |
11 | } |
12 |
13 | .container |
14 | { |
15 | padding-left : 18px ; |
16 | } |
Now let’s go to the FB canvas and see what is my page is showing. From the above example my canvas page shows the output like this:
Okay, if you want to add database, you just need to configure your application/config/databse.php file. Rest of the things are same.
Ahh ! It’s cool. I guess if I use any FW for FB application first I will check the volume of the application. I think there is no meaning to use FW for a small FB application.
No comments:
Post a Comment